Dearpygui - Menu Bar
03/25/2023, SatWith dearpygui, there are two ways to create a menu bar that displays within your main application window. One method of creating a menu bar is to use the "Viewport Menu Bar" which is the traditional menu bar with the fixed horizontal row of menu entries found near the top of your main applications window and the other is the "Menu Bar" which is a floating menu window inside your main application window.
The 'Menu Bar' contains 'Menu' entries, and the 'Menu' entries contain the 'Menu Items'. The 'Menu Items' can reside directly underneath the 'Menu Bar'.
Viewport Menu Bar
Below is the annotated code sample from the main documentation page.
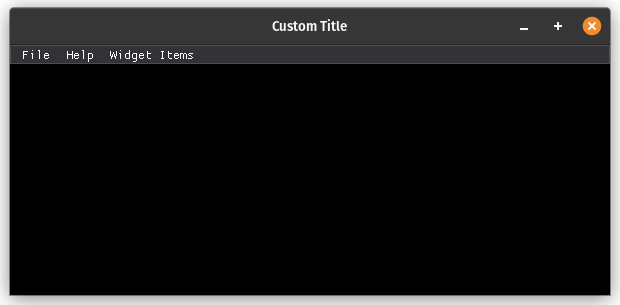
# Viewport Menu Bar
import dearpygui.dearpygui as dpg
def print_me(sender):
print(f"Menu Item: {sender}")
dpg.create_context()
dpg.create_viewport(title='Custom Title', width=600, height=200)
# Create the menu bar
with dpg.viewport_menu_bar():
# A top-level 'File' menu entry
with dpg.menu(label="File"):
dpg.add_menu_item(label="Save", callback=print_me)
dpg.add_menu_item(label="Save As", callback=print_me)
# Nested 'Settings' menu entry under 'File' with further nested
# 'Setting 1' and 'Setting 2' entries
with dpg.menu(label="Settings"):
dpg.add_menu_item(label="Setting 1", callback=print_me, check=True)
dpg.add_menu_item(label="Setting 2", callback=print_me)
# A top-level 'Help' menu item entry that triggers a callback upon clicking
dpg.add_menu_item(label="Help", callback=print_me)
# A top-level 'Widget Items' menu entry with items that can be added which
# are not a direct 'menu item'
with dpg.menu(label="Widget Items"):
dpg.add_checkbox(label="Pick Me", callback=print_me)
dpg.add_button(label="Press Me", callback=print_me)
dpg.add_color_picker(label="Color Me", callback=print_me)
# Application setup
dpg.setup_dearpygui()
dpg.show_viewport()
dpg.start_dearpygui()
dpg.destroy_context()
Menu Bar Example
The 'Menu Bar' example below is nearly identical to the example shown above except that the call to create the menu bar is now switched to a dpg.window
call. This 'window' appears as a floating widget element within your main application window.
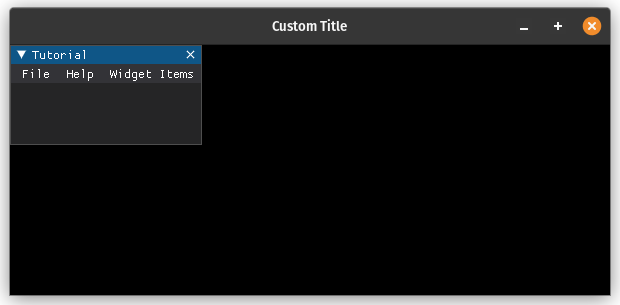
# Menu Bar Example
import dearpygui.dearpygui as dpg
dpg.create_context()
dpg.create_viewport(title='Custom Title', width=600, height=200)
def print_me(sender):
print(f"Menu Item: {sender}")
with dpg.window(label="Tutorial"):
with dpg.menu_bar():
with dpg.menu(label="File"):
dpg.add_menu_item(label="Save", callback=print_me)
dpg.add_menu_item(label="Save As", callback=print_me)
with dpg.menu(label="Settings"):
dpg.add_menu_item(label="Setting 1", callback=print_me, check=True)
dpg.add_menu_item(label="Setting 2", callback=print_me)
dpg.add_menu_item(label="Help", callback=print_me)
with dpg.menu(label="Widget Items"):
dpg.add_checkbox(label="Pick Me", callback=print_me)
dpg.add_button(label="Press Me", callback=print_me)
dpg.add_color_picker(label="Color Me", callback=print_me)
dpg.setup_dearpygui()
dpg.show_viewport()
dpg.start_dearpygui()
dpg.destroy_context()